2016-06-25 17:32:23 -04:00
|
|
|
itoa
|
|
|
|
====
|
|
|
|
|
|
|
|
[](https://travis-ci.org/dtolnay/itoa)
|
|
|
|
[](https://crates.io/crates/itoa)
|
2019-05-01 21:09:29 -04:00
|
|
|
[](https://docs.rs/itoa)
|
2016-06-25 17:32:23 -04:00
|
|
|
|
|
|
|
This crate provides fast functions for printing integer primitives to an
|
2019-05-01 21:09:29 -04:00
|
|
|
[`io::Write`] or a [`fmt::Write`]. The implementation comes straight from
|
|
|
|
[libcore] but avoids the performance penalty of going through
|
|
|
|
[`fmt::Formatter`].
|
2016-06-25 17:32:23 -04:00
|
|
|
|
2019-05-01 21:09:29 -04:00
|
|
|
See also [`dtoa`] for printing floating point primitives.
|
|
|
|
|
|
|
|
[`io::Write`]: https://doc.rust-lang.org/std/io/trait.Write.html
|
|
|
|
[`fmt::Write`]: https://doc.rust-lang.org/core/fmt/trait.Write.html
|
|
|
|
[libcore]: https://github.com/rust-lang/rust/blob/b8214dc6c6fc20d0a660fb5700dca9ebf51ebe89/src/libcore/fmt/num.rs#L201-L254
|
|
|
|
[`fmt::Formatter`]: https://doc.rust-lang.org/std/fmt/struct.Formatter.html
|
|
|
|
[`dtoa`]: https://github.com/dtolnay/dtoa
|
|
|
|
|
|
|
|
```toml
|
|
|
|
[dependencies]
|
|
|
|
itoa = "0.4"
|
|
|
|
```
|
2016-09-26 03:53:23 -04:00
|
|
|
|
2017-03-25 15:21:19 -04:00
|
|
|
## Performance (lower is better)
|
2016-06-25 17:35:57 -04:00
|
|
|
|
|
|
|
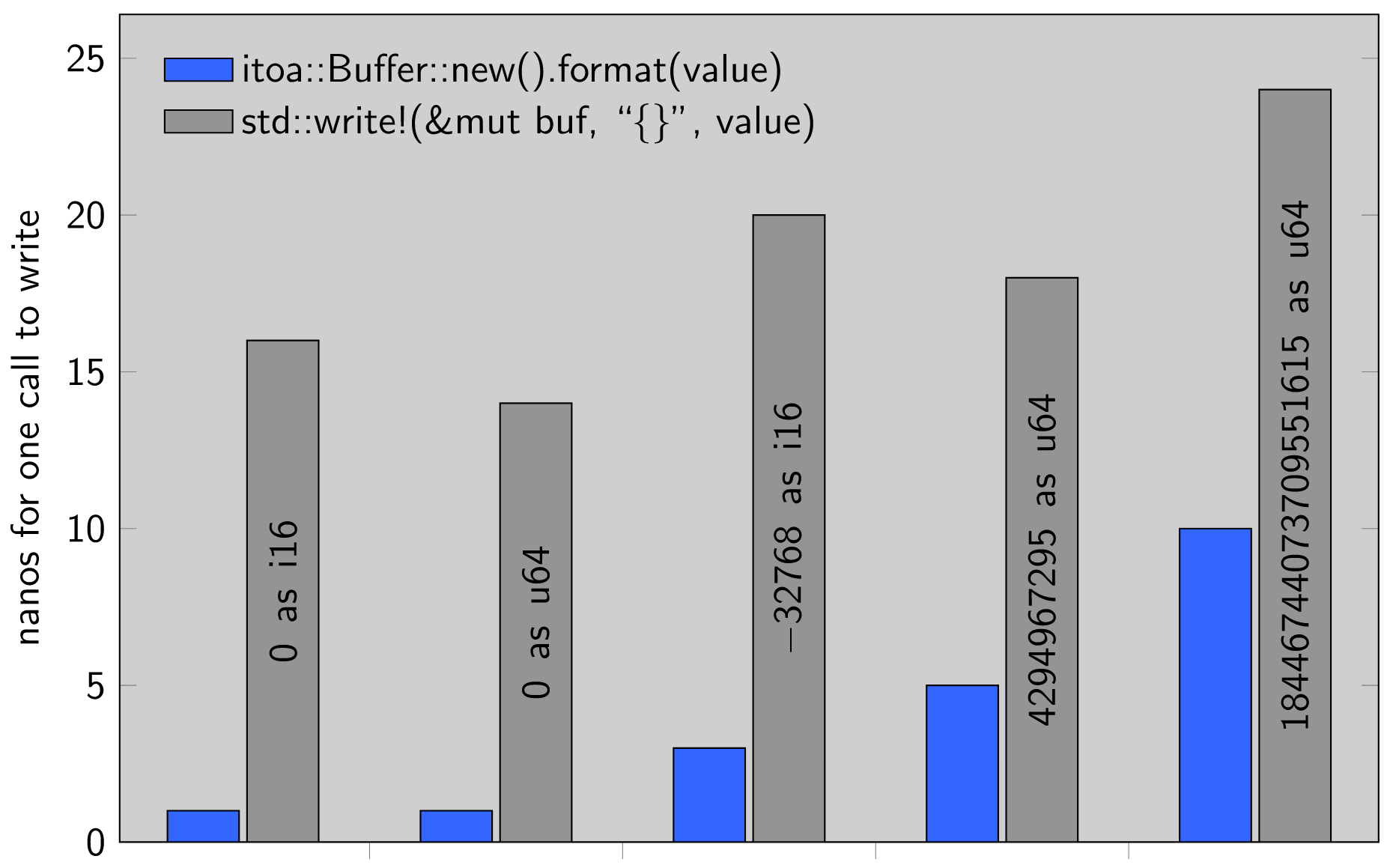
|
|
|
|
|
2019-05-01 21:09:29 -04:00
|
|
|
## Examples
|
2016-06-25 17:32:23 -04:00
|
|
|
|
|
|
|
```rust
|
2019-05-01 21:09:29 -04:00
|
|
|
use std::{fmt, io};
|
|
|
|
|
|
|
|
fn demo_itoa_write() -> io::Result<()> {
|
|
|
|
// Write to a vector or other io::Write.
|
|
|
|
let mut buf = Vec::new();
|
|
|
|
itoa::write(&mut buf, 128u64)?;
|
|
|
|
println!("{:?}", buf);
|
|
|
|
|
|
|
|
// Write to a stack buffer.
|
|
|
|
let mut bytes = [0u8; 20];
|
|
|
|
let n = itoa::write(&mut bytes[..], 128u64)?;
|
|
|
|
println!("{:?}", &bytes[..n]);
|
|
|
|
|
|
|
|
Ok(())
|
|
|
|
}
|
|
|
|
|
|
|
|
fn demo_itoa_fmt() -> fmt::Result {
|
|
|
|
// Write to a string.
|
|
|
|
let mut s = String::new();
|
|
|
|
itoa::fmt(&mut s, 128u64)?;
|
|
|
|
println!("{}", s);
|
|
|
|
|
|
|
|
Ok(())
|
|
|
|
}
|
2016-06-25 17:32:23 -04:00
|
|
|
```
|
|
|
|
|
2018-03-16 21:11:33 -04:00
|
|
|
The function signatures are:
|
2016-06-25 17:32:23 -04:00
|
|
|
|
|
|
|
```rust
|
2018-03-18 03:18:12 -04:00
|
|
|
fn write<W: io::Write, V: itoa::Integer>(writer: W, value: V) -> io::Result<usize>;
|
2018-03-16 21:11:33 -04:00
|
|
|
|
2018-03-18 03:18:12 -04:00
|
|
|
fn fmt<W: fmt::Write, V: itoa::Integer>(writer: W, value: V) -> fmt::Result;
|
2016-06-25 17:32:23 -04:00
|
|
|
```
|
|
|
|
|
|
|
|
where `itoa::Integer` is implemented for `i8`, `u8`, `i16`, `u16`, `i32`, `u32`,
|
2017-09-12 16:52:24 -04:00
|
|
|
`i64`, `u64`, `i128`, `u128`, `isize` and `usize`. 128-bit integer support is
|
|
|
|
only available with the nightly compiler when the `i128` feature is enabled for
|
|
|
|
this crate. The return value gives the number of bytes written.
|
2016-06-25 17:32:23 -04:00
|
|
|
|
2018-03-16 21:14:54 -04:00
|
|
|
The `write` function is only available when the `std` feature is enabled
|
|
|
|
(default is enabled).
|
|
|
|
|
2019-05-01 21:09:29 -04:00
|
|
|
<br>
|
2016-06-25 17:32:23 -04:00
|
|
|
|
2019-05-01 21:09:29 -04:00
|
|
|
#### License
|
2016-06-25 17:32:23 -04:00
|
|
|
|
2019-05-01 21:09:29 -04:00
|
|
|
<sup>
|
|
|
|
Licensed under either of <a href="LICENSE-APACHE">Apache License, Version
|
|
|
|
2.0</a> or <a href="LICENSE-MIT">MIT license</a> at your option.
|
|
|
|
</sup>
|
2016-06-25 17:32:23 -04:00
|
|
|
|
2019-05-01 21:09:29 -04:00
|
|
|
<br>
|
2016-06-25 17:32:23 -04:00
|
|
|
|
2019-05-01 21:09:29 -04:00
|
|
|
<sub>
|
2016-06-25 17:32:23 -04:00
|
|
|
Unless you explicitly state otherwise, any contribution intentionally submitted
|
2019-05-01 21:09:29 -04:00
|
|
|
for inclusion in this crate by you, as defined in the Apache-2.0 license, shall
|
|
|
|
be dual licensed as above, without any additional terms or conditions.
|
|
|
|
</sub>
|