diff --git a/README.md b/README.md
index 759be9d..2caf54b 100644
--- a/README.md
+++ b/README.md
@@ -3,41 +3,57 @@ itoa
[](https://travis-ci.org/dtolnay/itoa)
[](https://crates.io/crates/itoa)
+[](https://docs.rs/itoa)
This crate provides fast functions for printing integer primitives to an
-[`io::Write`](https://doc.rust-lang.org/std/io/trait.Write.html) or a
-[`fmt::Write`](https://doc.rust-lang.org/core/fmt/trait.Write.html). The
-implementation comes straight from
-[libcore](https://github.com/rust-lang/rust/blob/b8214dc6c6fc20d0a660fb5700dca9ebf51ebe89/src/libcore/fmt/num.rs#L201-L254)
-but avoids the performance penalty of going through
-[`fmt::Formatter`](https://doc.rust-lang.org/std/fmt/struct.Formatter.html).
+[`io::Write`] or a [`fmt::Write`]. The implementation comes straight from
+[libcore] but avoids the performance penalty of going through
+[`fmt::Formatter`].
-See also [`dtoa`](https://github.com/dtolnay/dtoa) for printing floating point
-primitives.
+See also [`dtoa`] for printing floating point primitives.
+
+[`io::Write`]: https://doc.rust-lang.org/std/io/trait.Write.html
+[`fmt::Write`]: https://doc.rust-lang.org/core/fmt/trait.Write.html
+[libcore]: https://github.com/rust-lang/rust/blob/b8214dc6c6fc20d0a660fb5700dca9ebf51ebe89/src/libcore/fmt/num.rs#L201-L254
+[`fmt::Formatter`]: https://doc.rust-lang.org/std/fmt/struct.Formatter.html
+[`dtoa`]: https://github.com/dtolnay/dtoa
+
+```toml
+[dependencies]
+itoa = "0.4"
+```
## Performance (lower is better)
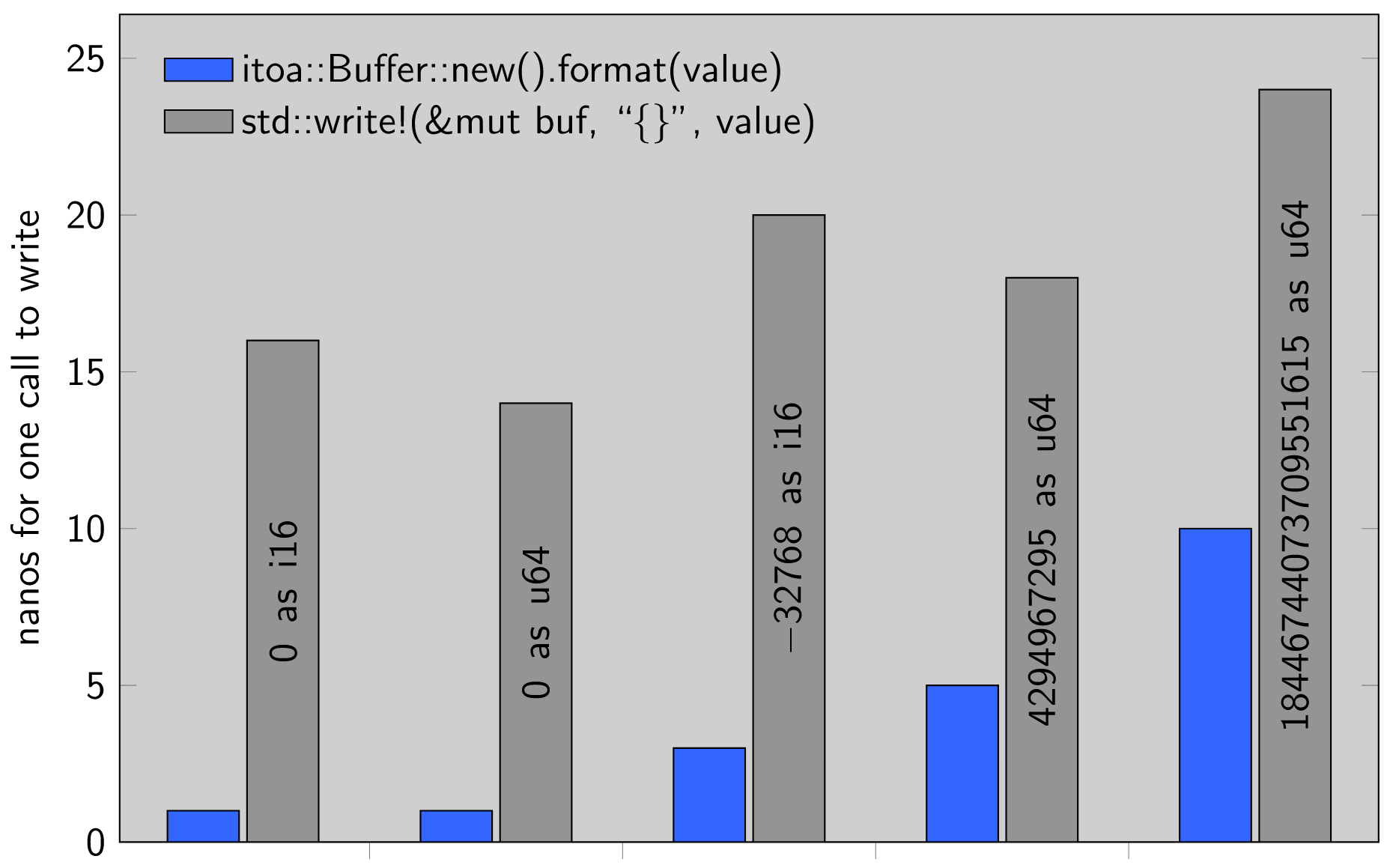
-## Functions
+## Examples
```rust
-extern crate itoa;
+use std::{fmt, io};
-// write to a vector or other io::Write
-let mut buf = Vec::new();
-itoa::write(&mut buf, 128u64)?;
-println!("{:?}", buf);
+fn demo_itoa_write() -> io::Result<()> {
+ // Write to a vector or other io::Write.
+ let mut buf = Vec::new();
+ itoa::write(&mut buf, 128u64)?;
+ println!("{:?}", buf);
-// write to a stack buffer
-let mut bytes = [b'\0'; 20];
-let n = itoa::write(&mut bytes[..], 128u64)?;
-println!("{:?}", &bytes[..n]);
+ // Write to a stack buffer.
+ let mut bytes = [0u8; 20];
+ let n = itoa::write(&mut bytes[..], 128u64)?;
+ println!("{:?}", &bytes[..n]);
-// write to a String
-let mut s = String::new();
-itoa::fmt(&mut s, 128u64)?;
-println!("{}", s);
+ Ok(())
+}
+
+fn demo_itoa_fmt() -> fmt::Result {
+ // Write to a string.
+ let mut s = String::new();
+ itoa::fmt(&mut s, 128u64)?;
+ println!("{}", s);
+
+ Ok(())
+}
```
The function signatures are:
@@ -56,27 +72,19 @@ this crate. The return value gives the number of bytes written.
The `write` function is only available when the `std` feature is enabled
(default is enabled).
-## Dependency
+
-Itoa is available on [crates.io](https://crates.io/crates/itoa). Use the
-following in `Cargo.toml`:
+#### License
-```toml
-[dependencies]
-itoa = "0.4"
-```
+
+Licensed under either of Apache License, Version
+2.0 or MIT license at your option.
+
-## License
-
-Licensed under either of
-
- * Apache License, Version 2.0 ([LICENSE-APACHE](LICENSE-APACHE) or http://www.apache.org/licenses/LICENSE-2.0)
- * MIT license ([LICENSE-MIT](LICENSE-MIT) or http://opensource.org/licenses/MIT)
-
-at your option.
-
-### Contribution
+
+
Unless you explicitly state otherwise, any contribution intentionally submitted
-for inclusion in itoa by you, as defined in the Apache-2.0 license, shall be
-dual licensed as above, without any additional terms or conditions.
+for inclusion in this crate by you, as defined in the Apache-2.0 license, shall
+be dual licensed as above, without any additional terms or conditions.
+